How well do you understand Vue.js templates? Can you create a dynamic and responsive user interface using them? Have you ever struggled when taking your Vue.js concept and turning it into a reality?
The process of conceptualizing and actualizing Vue.js templates has been a daunting task for many developers. As suggested by Laracasts (2020), developers deal with both the complexity of the Vue.js language itself and the difficulty of implementing the designs accurately. Hacker Noon (2019) also highlights that many developers struggle with the steep learning curve required to become proficient at Vue.js. From these challenges arises the need for an effective guide on Vue.js templates from inception to actualization.
In this article, you will learn about strategies that make the Vue.js template creation journey less cumbersome and more efficient. The article covers the different aspects that make a Vue.js template including data binding, computed properties, directives among others. It also provides practical steps on how to implement these aspects to create an effective Vue.js template.
By the end of the article, you should have a clear path to follow as you strive to turn your Vue.js concepts into user-friendly, dynamic, and responsive web pages. Whether you’re a beginner or well-versed with Vue.js templates, this article has something for you.
Definitions and Meanings: A Journey through Vue.js Templates
Vue.js is a popular Javascript framework used for developing user interfaces and single-page applications. It offers a simple and accessible way for web developers to build dynamic and interactive websites.
Templates refer to prewritten chunks of code that can be used as a starting point for certain programming tasks. In Vue.js, these templates are written in HTML, and they can be used to define how your Vue application will render on the webpage.
Concept to Creation in this context signifies the whole process of understanding a concept (or idea) and turning it into a functional, workable product. In this case, it refers to the journey of how one takes the idea of using Vue.js templates and actually creates a webpage or application using them.
Unveiling the Mystery: Setting the Stage with Vue.js Templates Concept
The Core Concept of Vue.js Templates
Vue.js templates are mark-up-like syntax that allows developers to declaratively render dynamic data into the DOM. It’s crucial to grasp that unlike in JavaScript, where a variable defines what a piece of code or function does, in Vue.js templates, a variable defines what the template will look like when rendered.
The Vue instance and the syntax of the Vue.js templates are tied together by the Vue compiler. When a Vue instance is created, it adds all the properties found in its data object to Vue’s reactivity system. When rendering, Vue creates a “watcher” for each property, which checks for changes. If a change is detected in any property, Vue automatically triggers a view update.
Vue.js uses HTML-based template syntax that allows developers to declaratively bind the rendered DOM to the underlying Vue instance’s data. All Vue.js templates are valid HTML that can be parsed by spec-compliant browsers and HTML parsers. Vue compiles the templates into Virtual DOM render functions, thereby combining the flexibility of complete JavaScript with the simple syntax of mustache-like placeholders.
Setting the Stage: Templating in Action
To better understand Vue.js templates’ inner workings, take a look at this simple yet comprehensive example:
“`js
var vm = new Vue({
el: ‘#example’,
data: {
message: ‘Hello world!’
}
})
“`
Here, ‘message’ is a property of the data object, and ‘#example’ is the Vue instance attached to an HTML element. We can render the ‘message’ data into the DOM using the double-curlies syntax:
“`html
{{ message }}
“`
When the Vue instance is created and attached to ‘#example’, the placeholder will be replaced with the value of ‘message’, rendering ‘Hello world!’ in the `
` tag.
A Vue.js template can involve quite a lot. Here are a few things you can do with it:
- Render text: You can use mustache-like syntax ‘{{ }}’ to render text.
- RAW HTML Output: The ‘v-html’ directive is used to output real HTML.
- Attributes: With the ‘v-bind’ directive, we can bind attributes like ids, classes, etc.
Armed with this understanding, one realizes that Vue.js templates serve as a foundational block for future manipulations and elaborations, thereby amplifying your productivity as a developer.
Diving Deeper: Translating the Concept of Vue.js Templates into Creation
Ever pondered how Vue.js templates go from an abstract idea into tangible, practical application? Vue.js templates are the backbone of any Vue.js application. Their primary function is to bind the Vue.js instance data to the DOM, rendering systematic, reliable, and responsive user interface. Their integration into an application allows seamless data reactivity, rendering visible changes onto the DOM as and when the data within the Vue.js instance alters.
The Core Concern
The meta-issues with Vue.js templates lie in the fundamental misunderstandings about their purpose and misconceptions about their capabilities. Some believe Vue.js templates are restricted, rigid and not as efficient as JavaScript functions, leading to their common abuse and incorrect implementation. Vue.js templates are not HTML but HTML-like, which means they look like HTML but don’t act exactly like it. They are declarative, instructing Vue.js on how the DOM should evolve in response to the changing Vue.js instance data without any decision-making processes required on the developer’s part.
Best Practices Illustrated
Diving into examples showcasing prudent practices with Vue.js templates, component composition marks the top of the list. Dividing an application into multiple, reusable components improves readability, maintainability, and scalability. Templates should be small and focused, ideally focusing on one specific thing. Avoid complex expressions in your templates. Apart from increasing the template’s payload, they significantly decrease its readability. Instead, computed properties or methods should be leveraged for complex expressions. Prop validation is another efficient practice, safeguarding against any potential bugs and errors by proactively managing the types of prop values accepted by various components.
A Closer Look: Exploring the Challenges and Triumphs in Vue.js Templates Creation
Are Your Vue.js Templates Serving Their Ultimate Purpose?
Vue.js templates work as the crucial medium for communication between JS logic and the HTML. Consequently, are your templates optimally designed to serve this function? It is essential to be aware of Vue.js’s powerful template syntax as it plays a significant role in building single-page applications. This syntax allows you to declaratively render data into the DOM using a simple template. Moreover, it lets you manipulate the DOM directly without having to manage the full component lifecycle and state. Thus, an evident understanding of how this syntax works helps in creating more efficient and scalable applications.
Conundrums Caused by Misconception and Misimplementation
Interestingly, a common problem faced by many developers is the misconception about Vue.js templates’ nature and functionality. Often, developers fail to properly differentiate between local and global registration of components leading to an unnecessary overhead and complicating the development process. Furthermore, wrongfully using Vue directives can result in highly coupled and brittle code, reducing the potential of reusing components. It’s noteworthy that Vue.js templates are not simply HTML. They can include conditionals, loops, and Reactivity API reference which, when misused, can lead to a severe performance downside and even potential security issues. Hence, precise knowledge about Vue.js templates and their best use can save you from such complications.
Transforming Visions into Viable Vue.js Templates
To unleash the full potential of Vue.js templates, developers should adhere to some best practices. Using a watch function or computed property to respond to changes to Vue instance data helps keep your data flow explicit and easy to debug. Component isolation, achieved by using single-file components, enables separation of concern and enhances code organization. An equally important practice is to use the Vue.js Style Guide which provides guidelines for avoiding errors, anti-patterns, and inconsistencies. For example, when v-for is used along with v-if, it may lead to performance implications and complex features. The Style Guide recommends always using a computed property rather than an in-template complex expression to maintain comprehensibility and performance. Furthermore, usage of Vue’s built-in transitions to make component entry, exit, and lists updates elegant can greatly enrich the end-user’s experience. These principles, once embedded in your everyday coding routine, can enable you to create more dynamic and powerful applications with Vue.js templates.
Conclusion
Have we truly discovered the potential that lies in Vue.js templates? This inquiry is not only compelling but also signifies the boundless growth and opportunities that technological advancements like Vue.js presents. The exploration from concept to creation has elucidated the flexibility and scalability of Vue.js templates. By iteratively going through this journey, we are bound to unravel the strengths and versatility of Vue.js. But even more significant is how this knowledge enables us to craft solutions that redefine user experience and pace up the progress of business efficiency and digital innovation.
We highly encourage you to join us in the next exploration by subscribing to this blog. Your support is instrumental as we unveil deeper insights and unravel more secrets behind successful web application development using Vue.js. Your comments and inquiries are extremely valued, as they lead us towards a more compelling and enriching exploration. Remember to remain connected so you can be the first to enjoy our soon-to-be-released blogs and tutorials. We are working relentlessly to ensure we offer you an exhilarating journey through the vast world of Vue.js templates.
Rest assured, there are more breathtaking releases on the way. It’s our intent to ensure that your journey is filled with value and benefit. The expansiveness of Vue.js and the potential that lies untouched excites us as we look beyond today. Every new release will not only expand your knowledge but also expose you to modern best practices and trends in Vue.js. We can’t wait to deliver these treasured nuggets. Before long, you’ll become an expert in leveraging Vue.js templates to create intricate web designs with ease. Enjoy the Vue.js journey, for a world of limitless potential awaits!
F.A.Q.
1. What is the basic concept behind Vue.js templates?
Vue.js templates are written in HTML that can be parsed by spec-compliant browsers and HTML parsers. They convert your Vue data and methods into HTML format that can be rendered into the DOM.
2. What methodologies are involved in creating Vue.js templates?
Creating Vue.js templates involves understanding the Vue instance, building a data model, implementing methods, conditionals, and loop structures. It also requires a firm grasp of Vue Directives, which are the core features of Vue.js templates.
3. What core features of Vue.js are most beneficial in template creation?
The most beneficial core features for template creation in Vue.js are directives. Directives such as v-if, v-else, v-show, v-bind, v-model, v-on and v-for allow for dynamic and interactive web pages.
4. How challenging is it to create Vue.js templates for beginners?
For beginners, creating Vue.js templates might seem a bit challenging initially. However, familiarity with HTML and basic JavaScript can ease the learning curve and make this task manageable.
5. Can Vue.js templates be integrated with other JavaScript libraries?
Yes, Vue.js templates can easily be integrated with other JavaScript libraries. This is beneficial as it allows for the creation of more dynamic and interactive web experiences.
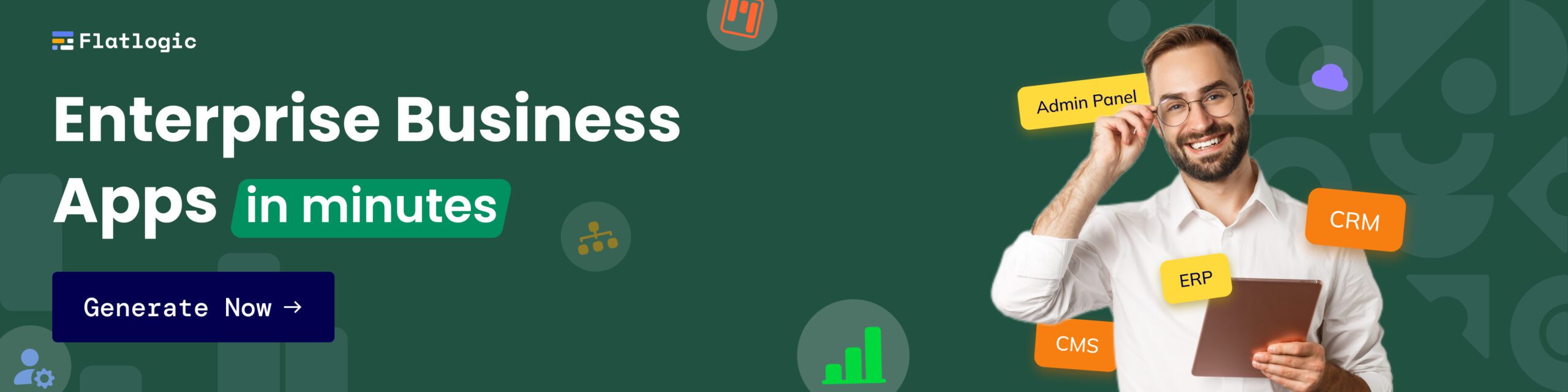